首页 > 代码库 > IOS自定义RadioGroup
IOS自定义RadioGroup
实现与android平台上相同的RadioGroup控件
//使用是只需要实现RadioGroup代理即可完成监听事件
[1].[图片] EBF2792E-4CB0-4318-B6B8-7CBA0E1E2DE5.png跳至 [1] [2]
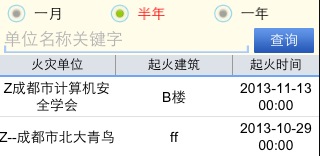
[2].[代码] Objective-C 跳至 [1] [2]
?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 | //RadioButton实现
#import <UIKit/UIKit.h> #import <QuartzCore/QuartzCore.h> #define RadioButton_WHeight 25 #define RadioButton_Space 5 typedef enum { CCRadioButtonAligmentLeft, //图片在左方 CCRadioButtonAligmentRight }CCRadioButtonAligment;
@protocol CCRadioButtonDelegate; @interface CCRadioButton : UIControl { @private CCRadioButtonAligment _aligment; CGSize _textSize; //使用layer减少内存消耗 CALayer *_imageLayer; CATextLayer *_labelLayer; } @property (nonatomic, strong) UIImage *image; //图片 @property (nonatomic, strong) UIColor *textColor; //字体颜色 @property (assign, nonatomic) id<CCRadioButtonDelegate> delegate; @property (nonatomic, getter = isChecked, setter = setCheck:) BOOL check; @property (nonatomic, strong) NSString *text;
- (id)initWithFrame:(CGRect)frame text:(NSString *)text aligment:(CCRadioButtonAligment)aligment isChecked:(BOOL)check; - (void)setCheck:(BOOL)check; @end
@protocol CCRadioButtonDelegate <NSObject>
- (void)onCheckedChanged:(CCRadioButton *)button;
@end
#import "CCRadioButton.h" #import "CCUITool.h"
@implementation CCRadioButton
- (id)initWithFrame:(CGRect)frame text:(NSString *)text aligment:(CCRadioButtonAligment)aligment isChecked:(BOOL)check { self = [super initWithFrame:frame]; if (self) { self.backgroundColor = [UIColor clearColor]; _check = check; _aligment = aligment; _text = text; /* 添加target */ [self addTarget:self action:@selector(valueChanged) forControlEvents:UIControlEventTouchUpInside]; //更具字的size 计算本控件的frame _textSize = [_text sizeWithFont:[CCUITool getDefaultFont:14] constrainedToSize:CGSizeMake(SCREEN_WIDTH, SCREEN_HEIGHT)]; self.frame = CGRectMake(self.frame.origin.x, self.frame.origin.y, RadioButton_WHeight + _textSize.width + RadioButton_Space, RadioButton_WHeight > _textSize.height ? RadioButton_WHeight : _textSize.height); } return self; }
- (void)drawRect:(CGRect)rect { if (!_imageLayer && !_labelLayer) { _imageLayer = [CALayer layer]; //计算_imageLayer 与_labelLayer 的frame _imageLayer.frame = _aligment == CCRadioButtonAligmentLeft ? CGRectMake(_textSize.width + 5, _textSize.height > RadioButton_WHeight ? (_textSize.height - RadioButton_WHeight)/2.0 : 0, RadioButton_WHeight, RadioButton_WHeight) : CGRectMake(0, _textSize.height > RadioButton_WHeight ? (_textSize.height - RadioButton_WHeight)/2.0 : 0, RadioButton_WHeight, RadioButton_WHeight); [self.layer addSublayer:_imageLayer]; _labelLayer = [CATextLayer layer]; _labelLayer.string = _text; _labelLayer.fontSize = 14; _labelLayer.frame = _aligment == CCRadioButtonAligmentLeft ? CGRectMake(0, _textSize.height > RadioButton_WHeight ? 0 : (RadioButton_WHeight - _textSize.height) / 2.0, _textSize.width, _textSize.height > RadioButton_WHeight ? _textSize.height : RadioButton_WHeight) : CGRectMake(RadioButton_WHeight+RadioButton_Space, (self.frame.size.height - _textSize.height)/2.0, _textSize.width, _textSize.height); [self.layer addSublayer:_labelLayer]; } / *重新切换图片,字体颜色(选中为红色)*/ _image = [UIImage imageNamed:_check ? @"radiobutton_checked" : @"radiobutton_normal"]; _textColor = _check ? [UIColor redColor] : [CCUITool getDefaultTextColor]; _imageLayer.contents = (id)_image.CGImage; _labelLayer.foregroundColor = _textColor.CGColor; }
- (void)valueChanged { if (!_check) { _check = !_check; if (_delegate && [_delegate respondsToSelector:@selector(onCheckedChanged:)]) { [_delegate onCheckedChanged:self]; } } }
- (void)setCheck:(BOOL)check { _check = check; [self setNeedsDisplay]; }
@end
//RadioGroup实现 #import <UIKit/UIKit.h> #import "CCRadioButton.h" typedef enum { CCRadioGroupOrientationHorizental, //RadioGroup水平放置 CCRadioGroupOrientationVertical }CCRadioGroupOrientation;
@protocol CCRadioGroupDelegate; @interface CCRadioGroup : UIView<CCRadioButtonDelegate/*实现RadioButton代理 */> { @private NSMutableArray *_buttonArray; CCRadioButton *_currentButton; } @property (assign, nonatomic) NSUInteger checkedIndex; @property (assign, nonatomic) id<CCRadioGroupDelegate> delegate;
- (id)initWithFrame:(CGRect)frame buttonTitle:(NSArray *)array orientation:(CCRadioGroupOrientation)orientation; - (void)checkIndex:(NSUInteger)index; - (CCRadioButton *)getCheckedRadioButton:(NSUInteger)checkId;
@end #import "CCRadioGroup.h" #import "CCUITool.h" #import "CCRadioGroupDelegate.h"
@implementation CCRadioGroup
- (id)initWithFrame:(CGRect)frame buttonTitle:(NSArray *)array orientation:(CCRadioGroupOrientation)orientation { self = [super initWithFrame:frame]; if (self) { _buttonArray = [NSMutableArray array]; CGFloat tempY = 0; for (int i = 0; i < array.count; i++) { CCRadioButton *radioButton = nil; switch (orientation) { case CCRadioGroupOrientationVertical: radioButton = [[CCRadioButton alloc] initWithFrame:CGRectMake(0, tempY, 0, 0) text:[array objectAtIndex:i] aligment:CCRadioButtonAligmentRight isChecked:NO]; tempY += 10; break; case CCRadioGroupOrientationHorizental: radioButton = [[CCRadioButton alloc] initWithFrame:CGRectMake(i * self.frame.size.width/array.count, 0, 0, 0) text:[array objectAtIndex:i] aligment:CCRadioButtonAligmentRight isChecked:NO]; break; } radioButton.tag = i; if (i == 0) { _currentButton = radioButton; } radioButton.delegate = self; [self addSubview:radioButton]; [_buttonArray addObject:radioButton]; } } return self; }
- (void)checkIndex:(NSUInteger)index { _currentButton = [_buttonArray objectAtIndex:index]; _currentButton.check = YES; _checkedIndex = index; }
- (CCRadioButton *)getCheckedRadioButton:(NSUInteger)checkId { return (CCRadioButton *)[_buttonArray objectAtIndex:checkId]; }
#pragma mark - CCRadioButtonDelegate - (void)onCheckedChanged:(CCRadioButton *)button { [_currentButton setCheck:NO]; _currentButton = button; [_currentButton setCheck:YES]; _checkedIndex = button.tag; if (_delegate && [_delegate respondsToSelector:@selector(onCheckedChanged:index:)]) { [_delegate onCheckedChanged:self index:button.tag]; } }
@end //RadioGroup代理 #import <Foundation/Foundation.h>
@class CCRadioGroup; @protocol CCRadioGroupDelegate <NSObject>
- (void)onCheckedChanged:(CCRadioGroup *)radioGroup index:(NSUInteger)checkId;
@end
//使用实例 NSArray *textArray = @[@"一月", @"半年", @"一年"]; CCRadioGroup *radioGroup = [[CCRadioGroup alloc] initWithFrame:CGRectMake(5, 2, SCREEN_WIDTH - 10, DEFAULT_HEIGHTLB) buttonTitle:textArray orientation:CCRadioGroupOrientationHorizental]; radioGroup.delegate = self;
//实现代理 - (void)onCheckedChanged:(CCRadioGroup *)radioGroup index:(NSUInteger)checkId { NSLog(@"点击了 -- %@", [radioGroup getCheckedRadioButton:checkId].text); } |
IOS自定义RadioGroup
声明:以上内容来自用户投稿及互联网公开渠道收集整理发布,本网站不拥有所有权,未作人工编辑处理,也不承担相关法律责任,若内容有误或涉及侵权可进行投诉: 投诉/举报 工作人员会在5个工作日内联系你,一经查实,本站将立刻删除涉嫌侵权内容。